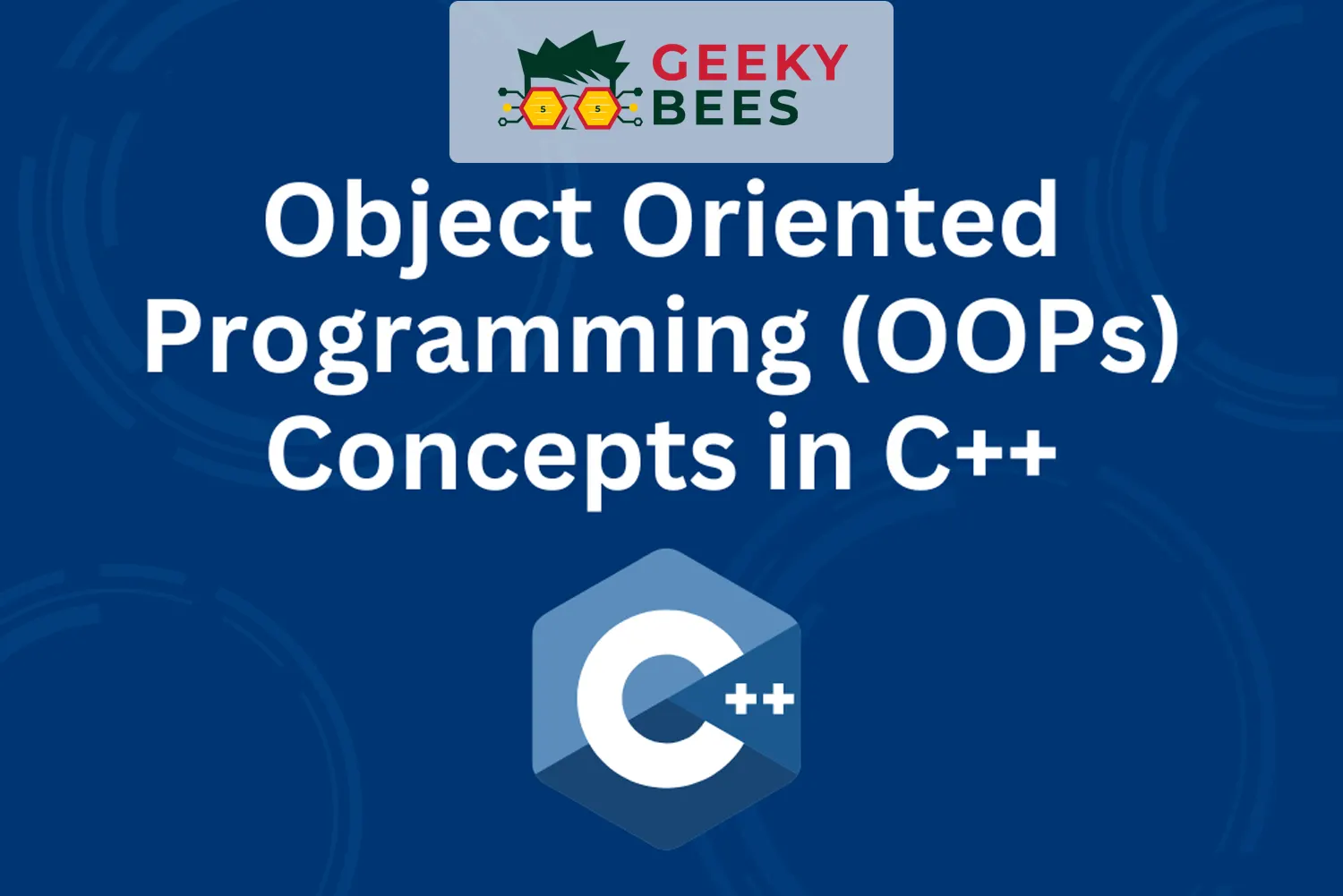
Course Description
Dive into the world of Object-Oriented Programming (OOP) with this comprehensive course designed for beginners and aspiring developers. Learn the foundational principles of C++ and explore advanced object-oriented design concepts that are widely used in modern software development. By the end of this course, you’ll be equipped to write clean, modular, and scalable code using C++.
Course Curriculum:
Module 1: Introduction to C++ and Object-Oriented Programming
- What is Object-Oriented Programming (OOP)?
- History and Applications of C++
- Setting up the C++ Environment
- First Program in C++
Module 2: Core Concepts of Object-Oriented Programming
- Classes and Objects
- Constructors and Destructors
- Member Functions and Data Members
- Static Members and Functions
Module 3: Object-Oriented Design Principles
- Encapsulation and Information Hiding
- Inheritance: Types and Usage
- Polymorphism: Compile-Time and Run-Time
- Abstraction and Interface Design
Module 4: Advanced Object-Oriented Programming in C++
- Overloading Operators
- Virtual Functions and Abstract Classes
- Exception Handling in C++
- Working with Templates
Module 5: Object-Oriented Design Patterns
- Common Design Patterns (Singleton, Factory, Observer)
- Writing Modular and Reusable Code
- Code Optimization Techniques
Module 6: Real-World Projects
- Building a Library Management System
- Designing a Basic Banking System
- Developing a Simple Game Using OOP Principles
Course Features:
- Interactive Lessons: Step-by-step explanations of C++ concepts.
- Hands-On Projects: Practical assignments to implement OOP principles.
- Quizzes and Assessments: Test your understanding of key concepts.
- Lifetime Access: Review lessons anytime, anywhere.